Berikut adalah contoh kode untuk program kasir kompleks yang memungkinkan pengguna untuk menambahkan produk, mencari produk, membeli barang, dan menampilkan total pembelian dengan menggunakan C++. Kode ini juga akan mengurangi stok produk secara otomatis setelah pembelian dilakukan.
C++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Product {
string name;
int price;
int stock;
};
vector<Product> products;
void addProduct(string name, int price, int stock) {
Product product;
product.name = name;
product.price = price;
product.stock = stock;
products.push_back(product);
}
Product* findProduct(string name) {
for (int i = 0; i < products.size(); i++) {
if (products[i].name == name) {
return &products[i];
}
}
return nullptr;
}
void buyProduct(string name, int quantity) {
Product* product = findProduct(name);
if (product != nullptr && product->stock >= quantity) {
int total = product->price * quantity;
cout << "Anda telah membeli " << quantity << " " << product->name << endl;
cout << "Total pembelian: " << total << endl;
product->stock -= quantity;
} else {
cout << "Produk tidak tersedia atau stok tidak mencukupi" << endl;
}
}
int main() {
bool running = true;
while (running) {
cout << "Menu:" << endl;
cout << "1. Tambahkan produk" << endl;
cout << "2. Cari produk" << endl;
cout << "3. Beli produk" << endl;
cout << "4. Keluar" << endl;
cout << "Pilih menu: ";
int choice;
cin >> choice;
switch (choice) {
case 1: {
string name;
int price, stock;
cout << "Nama produk: ";
cin >> name;
cout << "Harga: ";
cin >> price;
cout << "Stok: ";
cin >> stock;
addProduct(name, price, stock);
break;
}
case 2: {
string name;
cout << "Nama produk: ";
cin >> name;
Product* product = findProduct(name);
if (product != nullptr) {
cout << "Produk ditemukan" << endl;
cout << "Nama: " << product->name << endl;
cout << "Harga: " << product->price << endl;
cout << "Stok: " << product->stock << endl;
} else {
cout << "Produk tidak ditemukan" << endl;
}
break;
}
case 3: {
string name;
int quantity;
cout << "Nama produk: ";
cin >> name;
cout << "Jumlah: ";
cin >> quantity;
buyProduct(name, quantity);
break;
}
case 4:
running = false;
break;
default:
cout << "Pilihan tidak valid" << endl;
break;
}
cout << endl;
}
return 0;
}
C++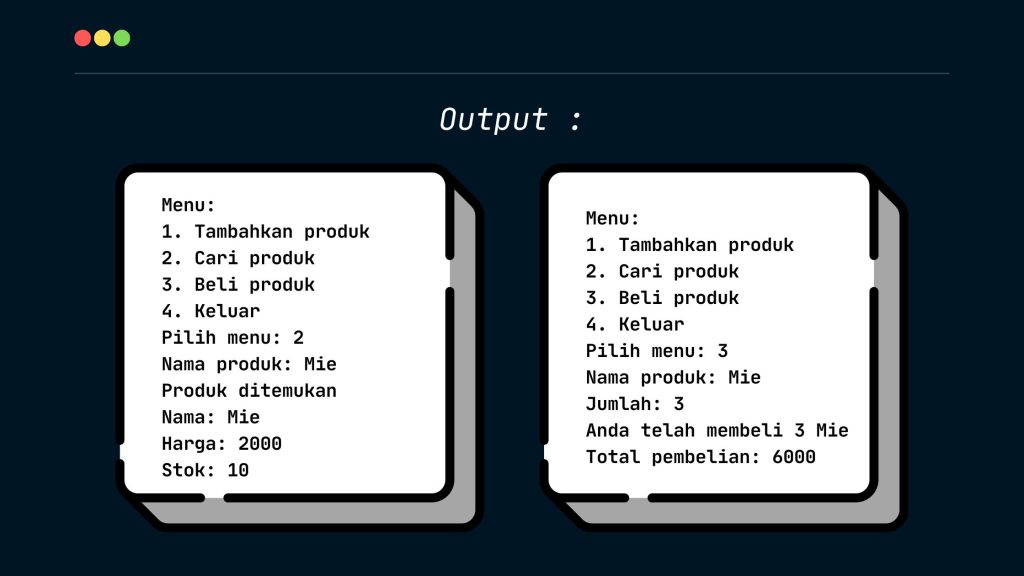
Baca juga:
Silakan gunakan kode di atas sebagai dasar untuk membuat program kasir kompleks yang sesuai dengan kebutuhan Anda. Jangan lupa untuk menyesuaikan dan mengubah kode sesuai dengan kebutuhan dan preferensi Anda.
Leave a Reply